CSCI 151 Lab 10: Binary Search Trees
Overview
In this lab, we will implement a few key methods of binary search trees.
Step 1: Setup
Download BinarySearchTree.java and BinaryTree.java, which we started in lecture.
Step 2: find (iterative)
Write a BinarySearchTree
method
public E find (E elt)
which finds the given element and returns it, or returns null
if the given element is not found. It should work via iteration, that is, using a while
loop.
Step 3: find (recursive)
Now write find
again, but using recursion: write
public E findR (E elt)
which finds the given element recursively.
Step 4: insert
Write a method
public void insert (E elt)
which inserts a new element into the proper place in a binary search tree.
Step 4: remove
Finally, write a method
public E remove (E elt)
These notes from lab explain how remove
should work:
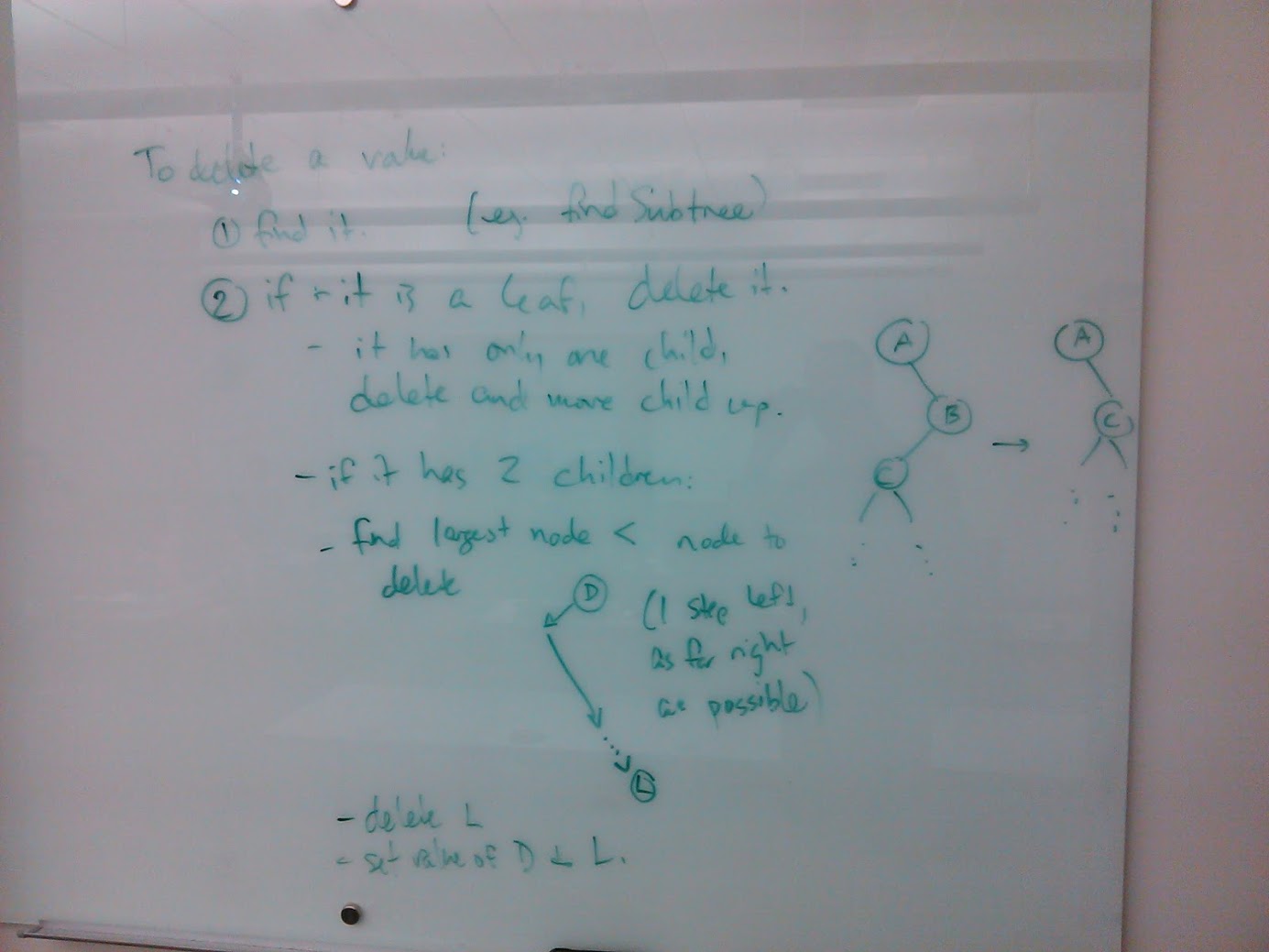